进程调度模拟程序设计
题目简单来说,就是模拟按优先数调度算法实现处理器调度的程序,详细题目如下:
进程调度模拟程序设计题目
做这个题目,比较方便的是用链表,而进程可以用如下结构体表示:
1 2 3 4 5 6 7
| struct PCB{ int name; PCB *next; int time; int prior; int status; };
|
在插入的时候,我们先按照大小查找到要插入的位置,即该节点(进程)的优先级比该位置的要大,就插入到该位置的前面:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| int PCBlist::insert(PCB p) { PCB *a = new PCB; a->name = p.name; a->time = p.time; a->prior = p.prior; a->status = p.status; if(!head->next) { head->next = a; a->next = nullptr; } else { PCB *b = head->next; PCB *c = head; for(; b; ) { if(a->prior >= b->prior) { c->next = a; a->next = b; break; } c = c->next; b = b->next; if(!b) { c->next = a; a->next = nullptr; }
} } return 0; }
|
所以在初始化中,我们就得到了一条完整的按照优先级排列的进程链表,我们只需要每次取出队首的进程,执行(优先级和时间-1),然后把它从这个链表中拿出,再重新按照优先级插入即可。当运行时间为0时,我们就可以不再重新插入它,而是直接输出状态为E,表示执行完毕:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| int run(PCBlist &T) { PCB *a = T.head; PCB *ne; a->next->time = a->next->time - 1; a->next->prior = a->next->prior - 1; ne = a->next; cout << "此时正在运行P" << ne->name << "程序" << endl; cout << "程序的运行时间还剩" << ne->time << endl; a->next = ne->next;
if(ne->time) T.insert(*ne); else{ cout << "进程P" << ne->name << "运行结束" << endl; cout << "------------------------------" << endl; cout << "进程名:P" << ne->name << ' '; cout << "优先级:" << ne->prior << ' '; cout << "剩余运行时间:" << ne->time << ' '; cout << "状态:E" << endl; cout << "------------------------------" << endl; } return 0; }
|
在每次run,也就是每次执行一个进程时,我们将链表输出,以查看当时的所以未执行完的进程的状态:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| int PCBlist::show() { PCB *a = head->next; for(; a; a = a->next) { cout << "------------------------------" << endl; cout << "进程名:P" << a->name << ' '; cout << "优先级:" << a->prior << ' '; cout << "剩余运行时间:" << a->time << ' '; cout << "状态:R" << endl; cout << "------------------------------" << endl; } return 0; }
|
1 2 3
| while(T.head->next) {run(T); T.show();}
|
下面放上全部的代码和执行结果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121
| #include <iostream> using namespace std; struct PCB{ int name; PCB *next; int time; int prior; int status; }; class PCBlist { public: PCB *head; PCBlist(); int insert(PCB p); int show(); }; PCBlist::PCBlist() { head = new PCB; head->next = nullptr; } int PCBlist::insert(PCB p) { PCB *a = new PCB; a->name = p.name; a->time = p.time; a->prior = p.prior; a->status = p.status; if(!head->next) { head->next = a; a->next = nullptr; } else { PCB *b = head->next; PCB *c = head; for(; b; ) { if(a->prior >= b->prior) { c->next = a; a->next = b; break; } c = c->next; b = b->next; if(!b) { c->next = a; a->next = nullptr; }
} } return 0; } int run(PCBlist &T) { PCB *a = T.head; PCB *ne; a->next->time = a->next->time - 1; a->next->prior = a->next->prior - 1; ne = a->next; cout << "此时正在运行P" << ne->name << "程序" << endl; cout << "程序的运行时间还剩" << ne->time << endl; a->next = ne->next;
if(ne->time) T.insert(*ne); else{ cout << "进程P" << ne->name << "运行结束" << endl; cout << "------------------------------" << endl; cout << "进程名:P" << ne->name << ' '; cout << "优先级:" << ne->prior << ' '; cout << "剩余运行时间:" << ne->time << ' '; cout << "状态:E" << endl; cout << "------------------------------" << endl; } return 0; } int PCBlist::show() { PCB *a = head->next; for(; a; a = a->next) { cout << "------------------------------" << endl; cout << "进程名:P" << a->name << ' '; cout << "优先级:" << a->prior << ' '; cout << "剩余运行时间:" << a->time << ' '; cout << "状态:R" << endl; cout << "------------------------------" << endl; } return 0; } int main() { PCBlist T; int n = 1; PCB a; int time; int prior; int status; for(; n < 6; n++) { a.name = n; cout << "请输入第" << n << "个进程的运行时间:" ; cin >> time; a.time = time; cout << "请输入第" << n << "个进程的优先级:" ; cin >> prior; a.prior = prior; a.status = 1; T.insert(a); } cout << "------------------------------" << endl; while(T.head->next) {run(T); T.show();} return 0; }
|
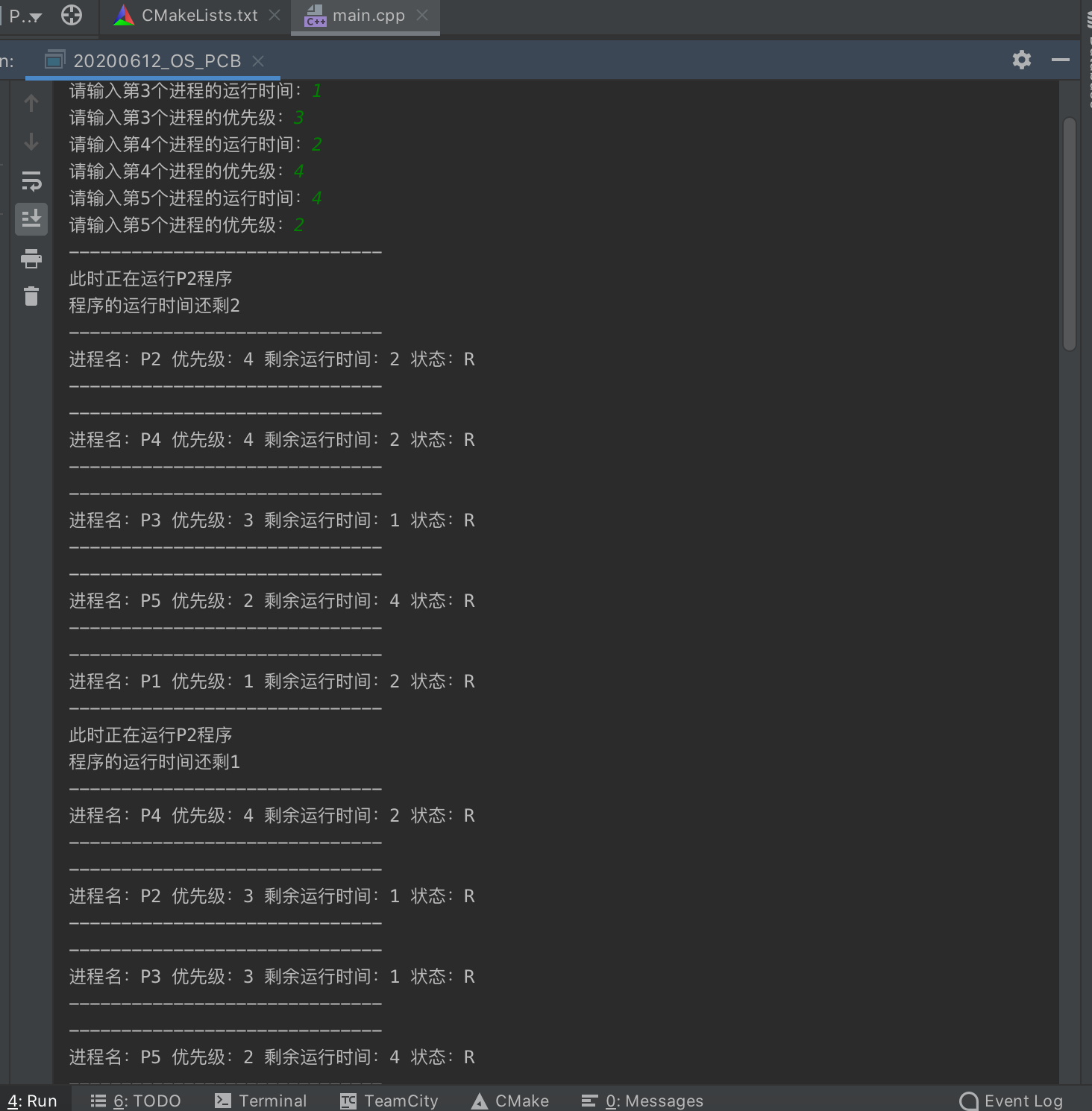
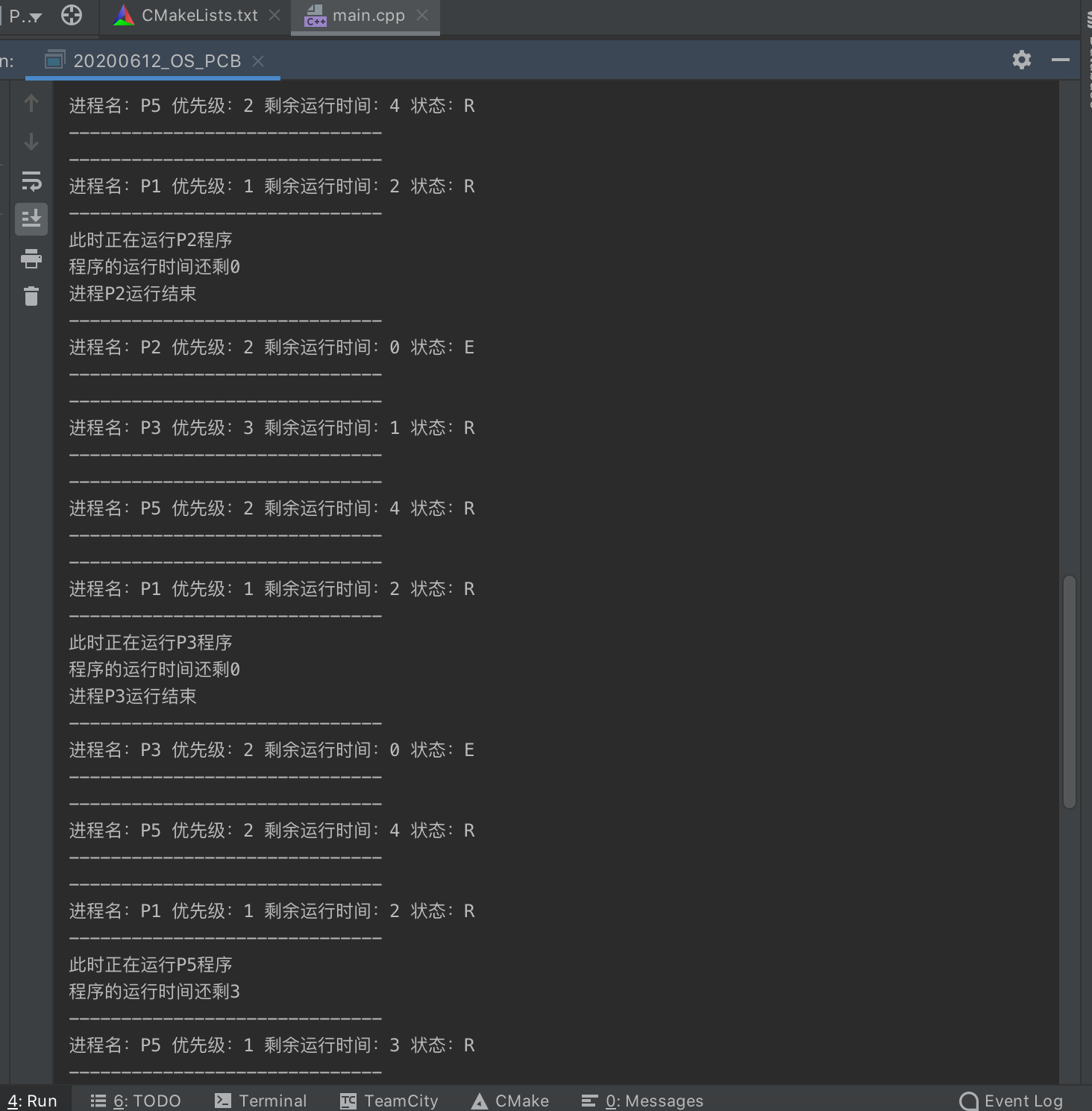
完