模拟文件系统程序
题目即为用C++模拟五个常用的DOS命令,create,MD,DIR,CD,DIRALL
详细题目:
模拟文件系统中的树型目录结构,并模拟实现常用Dos命令。如:Dir, Md, Cd, Rd, create filename等
1.创建目录结构。操作:选择一种树的存储结构,创建一个树。默认情况下,只含有一个根结点“#”。
模Create命令,创建新文件,添加到当前目录中。
格式:create filename filesize。操作:将文件作为结点加入到树中。
模拟MD命令,创建子目录,添加到当前目录中。
格式:MD directoryname。操作:将目录作为结点加入到树中。
模拟CD命令,进行父子目录见的切换。
格式:CD directoryname。操作:将当前状态改为dirctoryname目录。
模拟Dir命令,列出当前目录下的所有子目录名和文件名。
格式:Dir 。操作:遍历树结构,列出树结构中当前结点的孩子结点,并列出结点的性质(文件名,目录名)
模拟dir/all。列出当前目录下所有子目录和文件名。
其它:RD,CD,CD..,else提示:“Bad command”;
其它要求:在使用常用命令的过程中,不区分大小写。
提示:主程序用死循环,当用户敲入exit时,break,结束程序。
这个结构可以用树来简单模拟,但是树有多少个子节点是确定的,文件夹里有多少项目却是不确定的,所以我们可以使用子节点非常多的树结构去实现,这样是比较方便的,虽然子节点很多,但都是没有分配空间的指针,所以不会造成空间浪费。
树的结构
这里以五个子节点为例(即一个文件夹下最多放五个项目):
1 2 3 4 5 6 7
| struct BiNode { int type; string name; BiNode *onechild, *twochild, *threechild, *fourchild, *fivechild; }; typedef BiNode *BiTree;
|
首先我们要将其初始化:
1 2 3 4 5 6 7 8 9
| void init(BiTree &T) { T = new BiNode; T->onechild = nullptr; T->twochild = nullptr; T->threechild = nullptr; T->fourchild = nullptr; T->fivechild = nullptr; }
|
create函数
然后我们可以开始写create函数了,在这个结构里,因为我们只是展示下结构,没必要深究文件的结构,所以文件的结构和文件夹的数据结构是一致的,为了区分目录和文件的区别,我们节点里有一项type,当type为1时,说明是文件,是2时,说明是文件夹。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| void create(string name, BiTree &T) { BiNode *a; a = new BiNode; a->name = name; a->type = 1; if(!T->onechild) { T->onechild = a; cout << "创建" << name << "文件成功!" << endl; } else{ if(!T->twochild) { T->twochild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->threechild) { T->threechild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->fourchild) { T->fourchild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->fivechild) { T->fivechild = a; cout << "创建" << name << "文件成功!" << endl; }else{ cout << "该文件夹已满!" << endl; } } } } } }
|
当然MD操作和create是基本一致的:
MD函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| void MD(string name, BiTree &T) { BiNode *a; a = new BiNode; a->name = name; a->type = 2; if(!T->onechild) { T->onechild = a; cout << "创建" << name << "文件夹成功!" << endl; } else{ if(!T->twochild) { T->twochild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->threechild) { T->threechild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->fourchild) { T->fourchild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->fivechild) { T->fivechild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ cout << "该文件夹已满!" << endl; } } } } } }
|
DIR函数
接下来写DIR,DIR十分简单,只要把这个目录下的节点全部打印出来就好:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| int DIR(BiTree &T) { if(T->onechild) cout << T->onechild->name << ' '; if(T->twochild) cout << T->twochild->name << ' '; if(T->threechild) cout << T->threechild->name << ' '; if(T->fourchild) cout << T->fourchild->name << ' '; if(T->fivechild) cout << T->fivechild->name << ' '; cout << endl; return 0; }
|
CD函数
接下来是最难的CD,这里的CD只实现了最基本的功能,cd至子节点,或者cd至根,至于连跳这些,只需要对字符串处理分割就好了,这里不实现了。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| BiTree CD(BiTree &T, string dirname, BiNode *first) { if(dirname == "/") { return first; } if(T->onechild->name == dirname and T->onechild->type == 2) { return T->onechild; } if(T->twochild->name == dirname and T->twochild->type == 2) { return T->twochild; } if(T->threechild->name == dirname and T->threechild->type == 2) { return T->threechild; } if(T->fourchild->name == dirname and T->fourchild->type == 2) { return T->fourchild; } if(T->fivechild->name == dirname and T->fivechild->type == 2) { return T->fivechild; } cout << "No such file or directory" << endl; return T; }
|
这里的first是主函数里记录了T开头的一个指针:
1 2 3
| BiTree T; init(T); BiNode *first = T;
|
DIRALL函数
接下来是dirall,这就是简单的深度遍历了:
1 2 3 4 5 6 7 8 9 10 11 12 13
| int PreTraverse(BiTree T) { if (T == nullptr) return 0; cout << T->name; cout << ' '; PreTraverse(T->onechild); PreTraverse(T->twochild); PreTraverse(T->threechild); PreTraverse(T->fourchild); PreTraverse(T->fivechild); return 0; }
|
主函数
接下来是主函数,主函数是一个死循环,当输入exit时退出并结束:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| int main() { BiTree T; init(T); BiNode *first = T; while(1) { string cmd; cin >> cmd; if(cmd == "create" or cmd == "CREATE") { string name; cin >> name; create(name, T); } else if(cmd == "MD" or cmd == "md") { string name; cin >> name; MD(name, T); } else if(cmd == "DIR" or cmd == "dir") { DIR(T); } else if(cmd == "cd" or cmd == "CD") { string dirname; cin >> dirname; T = CD(T, dirname, first); } else if(cmd == "dirall" or cmd == "DIRALL") { PreTraverse(T); cout << endl; } else if(cmd == "exit" or cmd == "EXIT") { cout << "see you again!" << endl; return 0; } } }
|
演示和全部代码
接下来是演示当图片:
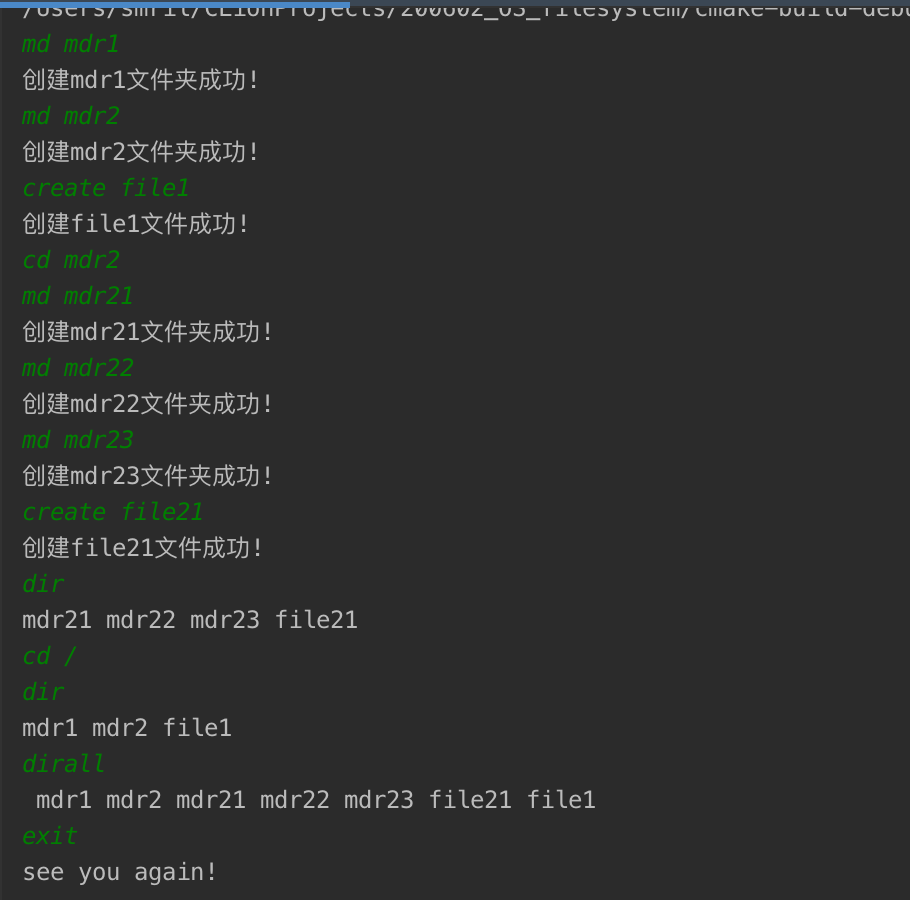
最后附上全部代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196
| #include <iostream> #include <string> using namespace std; struct BiNode { int type; string name; BiNode *onechild, *twochild, *threechild, *fourchild, *fivechild; }; typedef BiNode *BiTree; void init(BiTree &T) { T = new BiNode; T->onechild = nullptr; T->twochild = nullptr; T->threechild = nullptr; T->fourchild = nullptr; T->fivechild = nullptr; } void create(string name, BiTree &T) { BiNode *a; a = new BiNode; a->name = name; a->type = 1; if(!T->onechild) { T->onechild = a; cout << "创建" << name << "文件成功!" << endl; } else{ if(!T->twochild) { T->twochild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->threechild) { T->threechild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->fourchild) { T->fourchild = a; cout << "创建" << name << "文件成功!" << endl; }else{ if(!T->fivechild) { T->fivechild = a; cout << "创建" << name << "文件成功!" << endl; }else{ cout << "该文件夹已满!" << endl; } } } } } } void MD(string name, BiTree &T) { BiNode *a; a = new BiNode; a->name = name; a->type = 2; if(!T->onechild) { T->onechild = a; cout << "创建" << name << "文件夹成功!" << endl; } else{ if(!T->twochild) { T->twochild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->threechild) { T->threechild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->fourchild) { T->fourchild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ if(!T->fivechild) { T->fivechild = a; cout << "创建" << name << "文件夹成功!" << endl; }else{ cout << "该文件夹已满!" << endl; } } } } } } int PreTraverse(BiTree T) { if (T == nullptr) return 0; cout << T->name; cout << ' '; PreTraverse(T->onechild); PreTraverse(T->twochild); PreTraverse(T->threechild); PreTraverse(T->fourchild); PreTraverse(T->fivechild); return 0; } int DIR(BiTree &T) { if(T->onechild) cout << T->onechild->name << ' '; if(T->twochild) cout << T->twochild->name << ' '; if(T->threechild) cout << T->threechild->name << ' '; if(T->fourchild) cout << T->fourchild->name << ' '; if(T->fivechild) cout << T->fivechild->name << ' '; cout << endl; return 0; } BiTree CD(BiTree &T, string dirname, BiNode *first) { if(dirname == "/") { return first; } if(T->onechild->name == dirname and T->onechild->type == 2) { return T->onechild; } if(T->twochild->name == dirname and T->twochild->type == 2) { return T->twochild; } if(T->threechild->name == dirname and T->threechild->type == 2) { return T->threechild; } if(T->fourchild->name == dirname and T->fourchild->type == 2) { return T->fourchild; } if(T->fivechild->name == dirname and T->fivechild->type == 2) { return T->fivechild; } cout << "No such file or directory" << endl; return T; }
int main() { BiTree T; init(T); BiNode *first = T; while(1) { string cmd; cin >> cmd; if(cmd == "create" or cmd == "CREATE") { string name; cin >> name; create(name, T); } else if(cmd == "MD" or cmd == "md") { string name; cin >> name; MD(name, T); } else if(cmd == "DIR" or cmd == "dir") { DIR(T); } else if(cmd == "cd" or cmd == "CD") { string dirname; cin >> dirname; T = CD(T, dirname, first); } else if(cmd == "dirall" or cmd == "DIRALL") { PreTraverse(T); cout << endl; } else if(cmd == "exit" or cmd == "EXIT") { cout << "see you again!" << endl; return 0; } } }
|
完