实现登录验证
我们按照跳转的方式,将登录的用户直接跳转到主界面,即可实现登录功能,但是如果有人没有登录,但是他知道我们主界面的路由,他也一样可以进入主界面,所以我们通过登录验证的方法来限制这样的访问。
建立用户登录界面
首先我们按照以前的方式建立用户表,用户信息录入界面,然后我们建立一个叫做Login.php的控制器,在控制器里写入:
1 2 3 4 5 6 7 8 9 10 11
| <?php namespace app\keshe\controller; use think\Controller; use app\keshe\model\Yonghu; class Login extends Controller { public function login() { return $this->fetch(); } }
|
这一段很好理解,我们再去建立一个model文件就好了。接下来我们就是需要去写login.html文件。
在view下我们建立一个login文件夹,里面建立login.html文件,在html文件里写入:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no" /> <meta name="renderer" content="webkit"> <title>登录</title> <script src="/js/jquery.js"></script> <script src="/js/pintuer.js"></script> </head> <body> <div class="bg"></div> <div class="container"> <div class="line bouncein"> <div class="xs6 xm4 xs3-move xm4-move"> <div style="height:150px;"></div> <div class="media media-y margin-big-bottom"> </div> <form method="post" action="check"> <div class="panel loginbox"> <div class="text-center margin-big padding-big-top"><h1>后台管理中心</h1></div> <div class="panel-body" style="padding:30px; padding-bottom:10px; padding-top:10px;"> <div class="form-group"> <div class="field field-icon-right"> <input type="text" class="input input-big" name="yonghuhao" placeholder="用户号" data-validate="required:请填写账号" /> <span class="icon icon-user margin-small"></span> </div> </div> <div class="form-group"> <div class="field field-icon-right"> <input type="password" class="input input-big" name="mima" placeholder="密码" data-validate="required:请填写密码" /> <span class="icon icon-key margin-small"></span> </div> </div> <div class="form-group"> <div class="field"> <input type="text" class="input input-big" name="code" placeholder="填写右侧的验证码" data-validate="required:请填写右侧的验证码" /> <img src="{:captcha_src()}" alt="capcha" class="passcode" style="height:43px;cursor:pointer;" onclick="this.src=this.src+'?'"> </div> </div> </div> <div style="padding:30px;"><input type="submit" class="button button-block bg-main text-big input-big" value="登录"></div> </div> </form> </div> </div> </div>
</body> </html>
|
这里面包括了用户号,密码和验证码
验证用户是否正确
然后我们回去在控制器里写入一个方法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public function check() { $data = input('post.'); $user = new Yonghu(); $result = $user->where('yonghuhao',$data['yonghuhao'])->find(); if($result){ if($result['mima'] === $data['mima']){ session('yonghuhao',$data['yonghuhao']); } else{ $this->error('密码不正确'); } } else{ $this->error('用户名不存在'); exit; } if(captcha_check($data['code'])){ $this->success('登录成功!','login/index'); } else{ $this->error('验证码不正确'); } }
|
这段代码即验证我们输入的内容是否和数据库里的用户表信息相匹配。
其中有个session方法,这就是我们一会验证有无登录时做到。
我们输入一个正确的用户号和密码,点击登录,他就会提示登录成功:
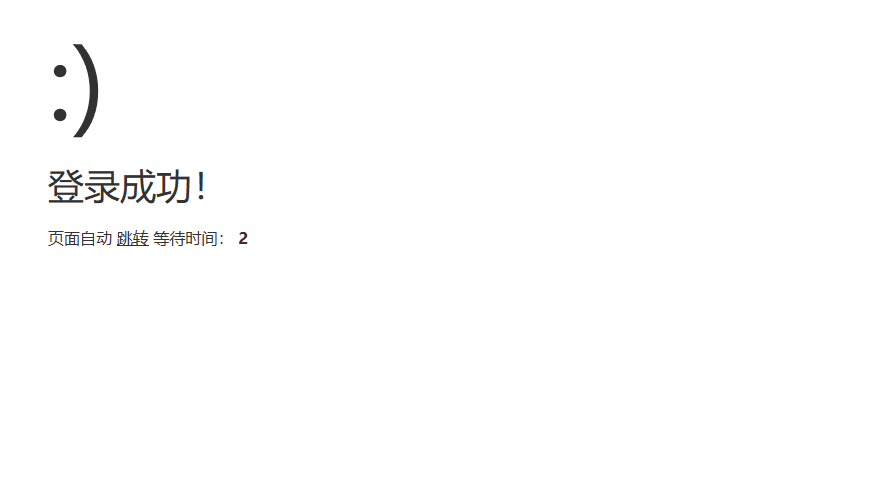
然后跳转到主界面:(主界面还未完善,中间的报错可以无视(´▽`))
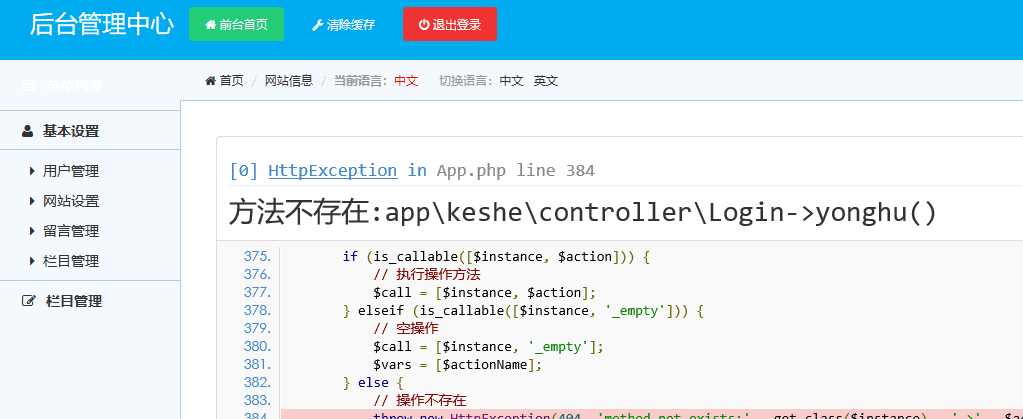
验证是否登录
但是现在我们直接输入主界面的路由,不用登录我们也能访问,所以我们要设置验证:
我们在新创建一个控制器base.php
1 2 3 4 5 6 7 8 9 10 11 12
| <?php namespace app\keshe\controller; use think\Controller; class Base extends Controller { public function _initialize() { if(!session('yonghuhao')){ $this->error('请先登录系统!','Login/login'); } } }
|
然后将我们的主界面上面更改为:
1 2 3 4 5 6
| <?php namespace app\keshe\controller; use app\keshe\controller\Base; use app\keshe\model\Yonghu as UserModel; use app\keshe\validate\Valyong as UserValidate; class Yonghu extends Base
|
这样,在执行主界面方法时,我们就会先去执行base.php, 在base.php里我们要验证session参数,当有这个参数,即为登录过后,我们才能访问主界面,否则,会提示请先登录,然后跳转到登录界面:
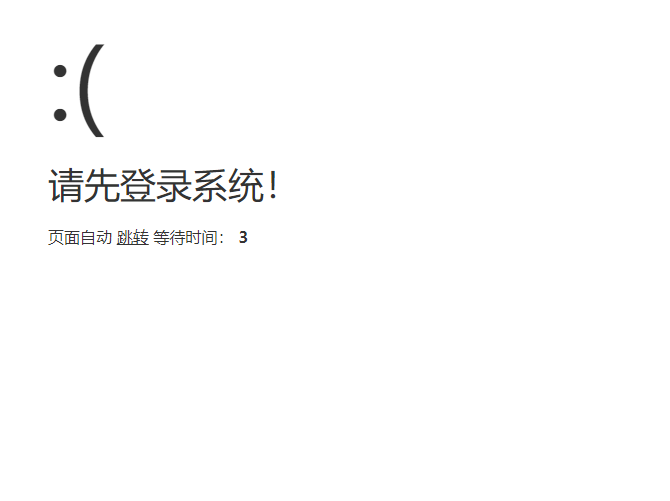